Before you start
Every GameArter SDK mode is designed for specific user-cases. Lite SDK is an ideal choice for developers who develop complete games.
- Continue only if following points are true:
- You want to connect the game with GameArter services (server saving, rewards, badges ...)
- Your game is complete. It does not need any web services like a shop etc.
Estimated time of SDK implementation: 2.5 hours. (2 hours of reading documentation, 0.5 hours of implementation) Please, follow the guide carefully. It will save your time and many future problems. Many Unity functionalities must be made via SDK (outgoing links, fullscreen to work properly with GameArter services).
- 1. Getting started
- 2. SDK Configuration
- 3. Working with SDK
- 4. Ready to use UI
- 5. Testing
- 6. Upload to GameArter
- 7. Game releases
1. Getting started
- Few points you need to have in mind during setting of SDK:
- User Accounts | User accounts are connected via SDK. You do not need to solve whether a user is logged in or not, or whether you have to display login box or not. This is done by SDK in background. You use same functions and calls for every user. SDK gets and saves data for logged players as well as for guests.
- Working with data | All important data must be saved in SDK. Do not use Local Storage, IndexedDb and others for saving important game data which may affect running of the game. These storages can be blocked or may crash. If data are not saved on GameArter server, there is no possibility to provide support for users in a case of any problem.
- SDK GUI displayed in the editor | SDK displays simple boxes simulating real more-complex windows displayed on the website. No box you see in the editor is actually displayed on the website, but functionality is same (icluding all callbacks and their state).
1.1. Download SDK package
Download latest version of GameArter SDK Unity package from GameArter SDK for HTML5 Package section.
1.2. Import GameArter SDK package to the game project
Importing GameArter SDK allows full work with SDK in own index.html file (editor mode) running on address file:// directly in the pc.
Importing GameArter's SDK consist of placing .css and .js file to index.html file.
After uploading final version to server, the index.html file will be automatically replaced for full GameArter's GamePlayer.
Be sure, GameArter's .css and .js file do not affect any game javascript or css (Only reserved var is "Garter"). Right import of GameArter's SDK require to keep following structure of index.html file:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<!-- Editor GamePlayer for testing (it will be automatically replaced after upload to gamearter) -->
<link rel="stylesheet" media="(min-width:480px)" href="https://www.gamearter.com/css/style-gp.css">
<link rel="stylesheet" media="(max-width:480px)" href="https://www.gamearter.com/css/mobile-wrapper.css">
<!-- GAME STYLES SPACE START-->
// Space for game styles
<!-- GAME STYLES SPACE END-->
</head>
<body>
<main role="main" id="main" class="main" style="height:100%;padding-left:0">
<div id="ga_game" style="height:100%;;position:relative">
<!------- GAME BODY SPACE START --------->
// Space for game body
<!--------- GAME BODY SPACE END ---------->
</div>
</main>
<footer role="contentinfo" class="footer-gp no-select">
<img src="https://data.pacogames.com/gplayer/branding/gamearter-uni.png" alt="Pacogames" width="184" height="18">
<a href="https://developers.gamearter.com/projects">Project Panel</a> |
<a href="https://developers.gamearter.com/docs/html5/sdk-overview.php">SDK Documentation</a>
</footer>
</body>
<script src="https://www.gamearter.com/gameplayer/html5/gamearter-gameplayer.js"></script>
<script src="https://www.gamearter.com/gameplayer/html5/gamearter-instance.js"></script>
<!-- GAME SCRIPTS SPACE START -->
// Space for game scripts
<!-- GAME SCRIPTS SPACE END -->
</html>
You can modify your own index.html file or extend following player prefabs - desktop-gameplayer.html, mobile-gameplayer.html - about your game lines.
In production, GameArter automatically run desktop or mobile gameplayer on basis of targeted device. This allow to deliver the best possible experience for these devices.
1.3. Fixing possible errors
- Game framework does not allow implementation of GameArter SDK by this way | Let us know your game framework / engine and we will make an extension of GameArter SDk for this framework / engine, if possible.
- Broken styling | This maight be affected either by a clash in GameArter's style with game style. There may be also different default style settings for running from localhost and server.
- No game displayed | Be sure that canvas (or game div) is displayed in visible area of screen. Game div must be inside GameArter's div element with id "ga_game".
- Need a help?GameArter Slack: @adm_vladimir / email - [email protected] /
2. SDK configuration
GameArter's SDK consists of 2 parts - client side and server side. The benefit of this solution is simple user, data and services management. The SDK communicates with server on basis of its project id.
2.1. Create a project
Create new html5 game project in projects section of GameArter system.
This is way you will get your project Id for communication with GameArter SDK.
SDK configuration will be set in 2 various panels. Panel 2.2 is designed for basic configuration, panel 2.3 allows extend basic configuration (2.2) about layer of additional features (adding badges, leaderboards, shops).
2.2. Project configuration
Purpose of project configuration is to set basic events on basis of which GameArter services will work. Every project can have up to 5 versions (1 production, 4 testnets) for development and testing purposes. Follow this documentation for creating your first project configuration.
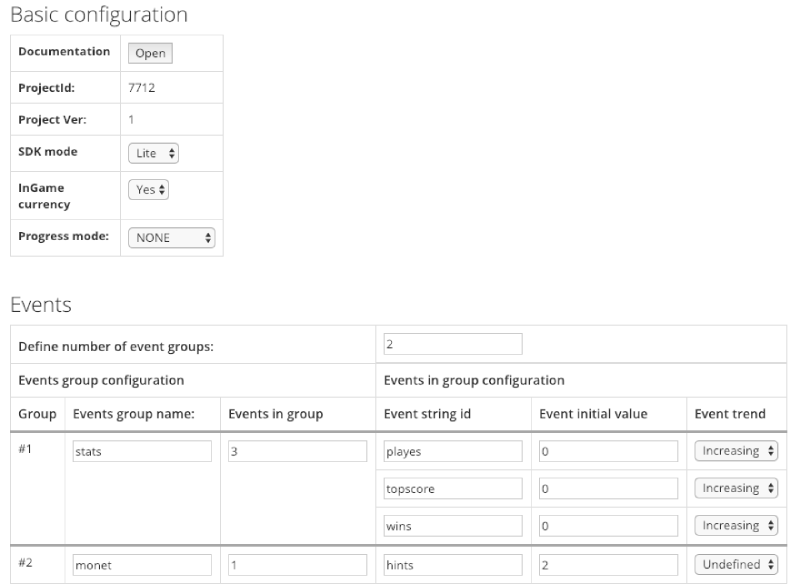
-
1
Documentation
A button opening knowledge base you are currently at. -
2
2.1 Project ID
Every project must have own unique ID. Project ID is a signature needed for communication with GameArter services. You got this id during creating your project in projects section of GameArter system. (point 2.1)
2.2 Project Version
For possibility of testing, GameArter provides to run up to 5 game versions. Every version has separated configuration and databases.
-
3
SDK mode
The mode, under which the SDK will run. Select Lite. -
4
In-game Currency
Select whether your game contains an in-game currency. If so, this currency will be used for GameArter currency exchange and in-game purchases.
-
5
Progress mode
SDK allows three types of counting user's game progress. Value of user's progress must be always saved in the SDK. This information is needed for displaying game info (progress, badges...) in the user's profile.
Current progress state can be displayed also in the game. If the game does not contain GUI for this value, there is possible to display user's progress with other user's data over GameArter_DashBoard prefab. If you want to use GameArter_DashBoard prefab, but without progress bar, switch its visibility to state "hidden".
- SDK | progress is being counted on a basis of received badges. (2 badges of 10 = progress 20%). You can display the progress in your own GUI, or via GameArter prefab GameArter_DashBoard prefab. If you want to use GameArter_DashBoard, but without the progress bar at the bottom, you can switch options of SDK Progress bar.
- Individual | SDK does not count progress, only holds its value. There is always need to send changes of user's progress into the SDK. Progress can be displayed in your own GUI, or via GameArter_DashBoard, see the point above.
- None | A game does not contain progress feature.
-
6
Smart Events
Before implementation, please read and try to understand Events purpose - In-game currency distribution. According to the event specification, make a plan, which game data will be saved as events and which as a different type of data.
Event explanation
Examples:
Event is an important variable connected with a feature (leaderboard) or a reward (achievements, currency reward), or with a shop for direct in-app purchases. Event example: kills in a shooter game , lap time in a racing game, number of gints in a logic game... Value of event can be increasing, decreasing or without defined trend. Events with defined trend can have attached unlimited number of badges on every event. There can be active also leaderboard above these events. Events with undefined trend are designed for direct connection with in-game shop. There is a possibility to work with events in many ways, events are written generally to allow anything a developer may need.-
"kills" event = total number of kills in a game
Initial value is 0 (new player). The number of kills can only grow in a time (= event with increasing trend). There will be a leaderboard connected with the event (most kills in the game). There will be badges - 1st kill, 10th kill, 100th kill... Leaderboard and badges are made and distributed by SDK. Developer only needs to keep best "kills" value in events = update the event with every kill. Required "kills" value for events is set on server (point 2.3). Then, once the value of the event will achieve the value of a badge, SDK will reward the badge to a user automatically. If a user sends a request to see a leaderboard, the SDK will create the leaderboard by comparing "kills" values of all users in the game. -
"bestLapTime" event = the lowset time achieved in a lap
Initial value is any not-null number. E.g. 120 (secs). Lower time is mostly better (= event with decreasing trend). There is a leaderboard for the time in the lap (made by SDK on basis of the best time of all users who completed the lap). There are 3 badges for the lap - gold / silver / bronze time. At the end of every lap, the developer checks time saved in the event "bestLapTime" and if current time is better (lower), he updates best achieved time in the lap. -
"maxAchievedMoney" event = a special help event allowing give own badge
This is a special event allowing to give a badge on basis of a dynamic variable. E.g. there is a need to add badges "Earned 1st dollar" and "Millionaire". The badges are connected with a currency which has dynamic value changeable in both directions. Then, if currency value will achieve a specific milestone, you can update event "maxAchievedMoney" and a user will be rewarded by a badge. -
"hints" event = number of available hints for a player.
Let's say a logic game will not contain any in-game currency, badges or leaderboards. All required functionality is implementation of in-game purchases. A user has certain number of hints to use (either one-time only, or every day) and in a case of need, he can buy more for GRT currency via native GameArter shop system.
Another possible event use-caseInitial values of events are changeable in web environment as well. Thanks to this option, events can be used also for game testing - If you set e.g. items to be unlocked on basis of some event value, you can unlock them directly from server without need of game rebuilding.
Define number of event groups | set number of groups in which you want to have events divided (better clarity)Event Group name - name of a group of eventsEvents in group - number of events in the group- Event string id | ID name of the event. Name in lowercase letters, without spaces. this is string ID over which you will get and set values for the events.
- Event Initial value | initial value of the events. (probably 0 for increasing trend). Any number > 0 you consider as optimal for decreasing trend. Any number >= 0 for undefined trend.
- Trend | select, whether the event has increasing trend (value number is better (kills), or decreasing (lower number is better (best time of the lap), or undefined (value can be changed via in-game shop - e.g. number of hints)
-
"kills" event = total number of kills in a game
Once you are done, save your configuration by button "Save configuration". (There is possibility to update this configuration also backward from panel 2.3)
2.3 Services configuration
Services configuration is for files management, project versions management and adjusting data filled in the project configuration and for adding additinal SDK features as badges, leaderboards and in-game purchases. Services configuration is manageable via the project panel. Project configuration is still available for option of updates under button "Export conf".
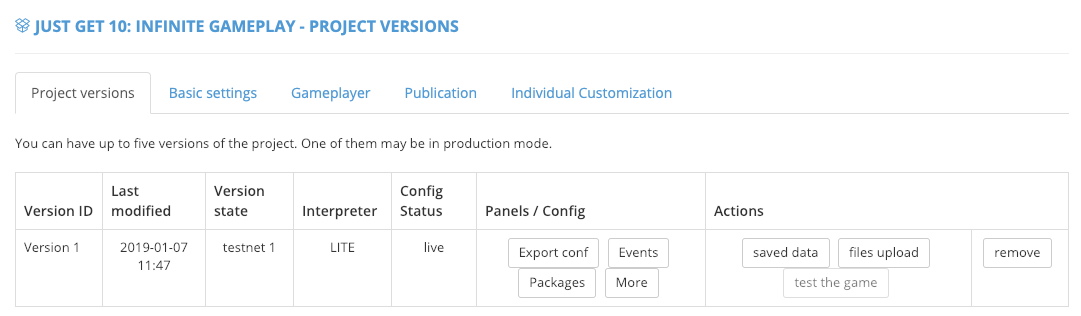
- Rows / Buttons explanation:
- Version ID | project version
- Last modified | Last modification in the version configuration
- Version State | project mode - testnet / production
- Interpreter | interpreter under which the project version runs
- Config status | Status of data state - draft / pending (= waiting for authorization by admins) / live (= approved,in production)
- Export conf buttonn | Opens project configuration (panel 2.2)
- Events button | Opens events configuraion (= rewards, badges, leaderboards)
- Packages button | Opens packages configuraion (= ingame purchases)
- More button | Opens configuraion of additional features
- Saved data button | Opens environment for reading / changing data of logged (developer) account in the game
- Files upload button | Opens environment for uploading game files
- Test the game button | Opens game in a new tab
- Remove button | Removes project version
-
1
Smart Events configuration
- Server-side contains all defined events. If not, contact support.
EventDetail- Event ID | non-changeable string ID. All data you will fill for the event are associated with this ID. If you change its name / remove the ID, all associated badges and leaderboards will be lost. Do not change this, if it is not necessary. If so, contact support for possibility to convert users' data below the new event ID.
- Leaderboard | Checkbox for activation of leaderboards for the event. SDK automatically creates leaderboards for the event. There are leadeabords created with filters 1 day / 1 week / 1 month / all-time. Trend of leaderboards is intended by event trend.
- Trend | Trend of event. From safety reasons, there is no possibility to manipulate with events in both directions. An event can only grow (increasing trend) or fall (decreasing trend). Usage: increasing trend - total number of kills, number of opened maps. Decreasing trend - best time of a lap.
- Initial Value | Initial value of event. By this, you can overwrite initial value set in the game. This value is returned to all new players. Default value for events with increasing trend = 0. Default value for events with decreasing trend - none, need to be set.
If availability of some item depends on a state of event value, this is way you can set the item property for new users without need of rebuilding game, directly on one click from server. - Reward Cx | Reward for occured event in a currency depending on a native game currency. (Full SDK = GRT, Lite SDK = GRT/local game coins on basis of local game coins existence). Reward is for a change about one unit. Examples:
- kill event - a coins reward for 1 kill
- lap of a round - better time lap about 0.2sec → reward per 1 unit (=1sec) = 1coin → 0.2*1 = 0.2 coin reward.
- Reward Exp | available for Full SDK mode only. Works same as Reward cx, only the reward is in game experience. On basis of achieved experience in the game by users, there is possible to automatically manage items - unlock them for using (see more in items configuration). This feature allows to create basic game design.
Add badge- Badge name | Name of a badge you want to add (e.g. 100 kills)
- Event value | Event value on achieving which the badge will be received (= 100)
- Coin reward | Received coin reward for received badge.
- Exp reward | Received coin reward for received badge. (full SDK only)
- Dx reward | Received diamonds for received badge. (rewards disabled currently)
- img url | image of a badge. Fill name of image file. If you do not have an image for the badge, let it be.
Event Badges List | list of created badges. You can adjust already defined badges here. If your game is already released and badges are rewarded, and you want to change value for a received badge, please contact support. Badges of all players will have to be recounted.
Badge Collections | Group more badges to a defined collection. Max number of collections is three. E.g. Character, car, others. Badge collections do badge listing in GUI clearer.
Saving events configurationFilled events must be saved. There are 2 options of saving. "Save as concept" saves the changes as a concept only - these saves are not visible from game. If you want to save the data and have access to them from a game, save them via "Save to production" button. If you do bigger changes for a project in production state "live", there is a need of approval by administrator. It is to prevent clashes which would lead to possible game crashes after changing values for badges, eventIDs and so on.
-
2
Packages configuration
Setting of packages for exchanges between game currency and GameArter currency.
- COMMODITY | commodity for exchange for GRT - game currency / certain event with undefined trend
- PUBLIC_NAME | commodity name visible in the shop.
- ACTION | buy / sell selected commodity for GRT.
- PACKAGE_IMAGE | image for the package offer. (if remains empty, default img will be used)
- VALUE_CHANGE | Value of commodity for certain amount of GRT
- CURRENCY | GRT = shared gamearter currency
- DISCOUNT | Displayes Discount span
Just press "save all events" button, that's all.
3. Working with SDK
3.1. SDK initialization
For possibility to connect and use GameArter SDK, there is need to create a new GameArter SDK instance.
var GameArterSdk = new GamearterInstance({
projectId:0, // Insert your project id here
projectVersion:1, // Insert your project version here
sdkMode:"L", // Use SDK in Lite mode
sdkVersion:"2.0" // use SDK ver 2.0
}, function(err){ if(err) console.error(err); } );
Callback function (attribute 2) is optional parameter via which you can be informed about errors occured during SDK initialization (e.g. SDK is already initialized, different projectId than expected projectId ... ).
During initialization, by adding other parametres there is possible to adjust SDK behavior. Following table display other options:
System options
key | value | default value | description |
---|---|---|---|
notifications | boolean | true | Displayes notification related to SDK ("Loading data", "saving data", "Badge XY received" ...) in top left corner. |
autoSaving | boolean | true | Automatically saves event values in certain frequency. (manual saves of game data are still required) |
editor options
key | value | default value | description |
---|---|---|---|
debug | boolean | true | Prints additional informations to console |
prerollAd | boolean | false | Activates preroll ad |
minAdTime | num [miliseconds] | 1000 | Sets minimum time between ads to GameArter's ad manager. (1000 = 1s) |
Example of creating SDK instance with additional options:
var GameArterSdk = new GamearterInstance({
projectId:0, // Insert your project id here
projectVersion:1, // Insert your project version here
sdkMode:"L", // Use SDK in Lite mode
sdkVersion:"2.0", // use SDK ver 2.0
notifications: true, // enable SDK notification
autoSaving: true, // enable autosaving
developmentMode: {
debug: false, // do not print to console
prerollAd: true, // display preroll ad
minAdTime: 60000 // set minimum time between ads at 1 minute
}
}, function(err){ if(err) console.error(err); } );
At the example above, SDK functions are available on path GameArterSdk.I. ... . Rest of the documentation will work with GameArterSdk as the var of GameArter instance.
3.2. SDK Listeners installation
In general, listeners are processed by callback function of calls made to the SDK. This sections is related to listeners for external events like SDK initialization or rewards to player (defined on server, not in the game).
Installation of listeners
GameArterSdk.I.AddExternalCbListener(A, B, C );
where:
- A: Event the listener is set for
- B: Function into which is sent information once the event occur
- C: Callback function informing about success / fail (optional)
Available external listeners
eventKey | attribute value | description |
---|---|---|
SdkInitialized | - | It is called once GameArter SDK is fully initialized (all calls and services are available) |
CurrencyUpdate | currency value | It is called on external currency change (e.g. via shop or reward mechanism). There is need to update displayed currency value in game UI on this event. |
EventExternalUpdate | stringId name of updated event, eventValue | It is called on external event change. Usually update of event with undefined trend via shop (see in-game purchases) |
ReceivedBadges | Array list of received badges | It is called once a user reaches conditions for badges in services configuration (point 2.3). Badges are rewarded automatically as well as basic notification is displayed via GameArter's notification module, if enables (See point 3.1). This callback is designed for opportunity to display own game UI actions / sounds on receiving badges. |
PossibleGameExit | - | It's called when SDK recognizes risk of escaping the game (e.g. closing page tab). This may be last opportunity to save game porgress. |
UserChanged | - | It's called when a user changes his identity - switching between user accounts - playing as a guest or selected logged user |
PWAState | - | Progressive Web App state. It's called when progressive web app state is being changed. -> enabled = display install game as app button | -> disabled = hide install game as app button. Button install game as app must be hidden in default. See more in section 3.15 - Game as App. |
Example of listeners installation for external SDK events
// Listeners
GameArterSdk.I.AddExternalCbListener("SdkInitialized", _GameArterSdkInitialized, function(err,res){ console.log(err,res); });
GameArterSdk.I.AddExternalCbListener("CurrencyUpdate", _CurrencyUpdate, function(err,res){ console.log(err,res); });
GameArterSdk.I.AddExternalCbListener("EventExternalUpdate", _EventValueExtUpdate, function(err,res){ console.log(err,res); });
GameArterSdk.I.AddExternalCbListener("ReceivedBadges", _ReceivedBadges, function(err,res){ console.log(err,res); });
GameArterSdk.I.AddExternalCbListener("PossibleGameExit", _PossibleGameExit, function(err,res){ console.log(err,res); });
GameArterSdk.I.AddExternalCbListener("UserChanged", _GameArterSdkInitialized, function(err,res){ console.log(err,res); });
GameArterSdk.I.AddExternalCbListener("PWAState", _PwaState, function(err,res){ console.log(err,res); });
// Listeners functions
function _CurrencyUpdate(value){ console.log("_CurrencyUpdate: ", currencyValue); }
function _EventValueExtUpdate(eventName, eventVal){ console.log("_EventValueExtUpdate: ", eventName, eventVal); }
function _ReceivedBadges(badgesListArray){ console.log("_ReceivedBadges: ", badgesListArray); }
function _PossibleGameExit(){ console.log("_PossibleGameExit: ", state); }
function _GameArterSdkInitialized(){ console.log("--- SDK INITIALIZED !!! -→ load the game ---"); }
function _PwaState(state){ console.log("PWA:",state); }
NOTE: From security reasons, GameArterSdk must be used within a scope (e.g. in function, class, or prototype) to prevent its global accessability.
Possible start implementation:
- Screen / box "Loading"
- Wait for "SdkInitialized" event.
- Set game - data, UI...
- Remove "Loading screen (point 1) and let a user play"
3.3. Accessing SDK data
-
1
Individual Game Mode
There's a possibility you require to run the game in individual game mode on certain website or e.g. for testing. By using individual game mode features, you can set individual mode the game will run in for every website the game is published on. SDK will return you after its initialization number of mode which you will assign to a certain website. Then, you can simply set data with which the game should run according the mode number.
Get mode number under which the game should run:
var individualGameModeNumber = GameArterSdk.I.IndividualGameMode(); -
2
Browser name
WebGL games have often different performance in various browsers. With knowledge of a browser a game is running in, there is a possibility for optimization of disabling features for certain browsers. E.g. car destructions for Chrome.
var browserName = GameArterSdk.I.GetBrowserName();
3.4. User data
Requests for interaction with user's data.
-
1
Accessing user data
Folowing calls will return information about the user. It is read-only data.
copyvar userNick = GameArterSdk.I.UserNick(); // user's nickname or Guest#randomNumber var userLang = GameArterSdk.I.UserLang(); // default language a user has set on pacogames // ar, cs, de, en, es, fr, pl, pt, ru, tr var userCountry = GameArterSdk.I.UserCountry(); // country shortcut the user is connected from var gameProgress = GameArterSdk.I.UserProgress ()); var loggedUser = GameArterSdk.I.IsLoggedUser(); // returns true, if user is logged in var userImageUrl = GameArterSdk.I.UserImage (); // returns user's profile img as a texture () [1]); var userRankName = GameArterSdk.I.UserRankName(); // returns profile rank of a user
-
2
User's game progress
SDK allows three types of counting game progress. Their configuration was in game-side configuration (section 2.2). No matter what type you have selected (if your game contains a tracker of progress), the progress value must be saved in SDK. Only this way, a user can see all games he is active in at his profile.
Game progress is in number set < 0, 100 > (in percentages). Do not use values 0-1 from a reason of float accuracy.- Getting user's game progress | var userPgrogress = GameArterSdk.I.UserProgress();
Returns value of progress.
- Setting user's game progress | GameArterSdk.I.UserProgress(absProgress);
If the game uses individual progress mode, you must inform SDK about current user's progress.
- Getting user's game progress | var userPgrogress = GameArterSdk.I.UserProgress();
3.5. Accessing events
Events are divided for holding the best achieved values. Events are also a way to add rewards, badges, leaderboards, or in-game purchases for event values.
-
Get | GameArterSdk.I.Event (eventNameId);
returns value of required event
-
Update (recommended) | GameArterSdk.I.Event (eventNameId, change);
updates value of required event and returns its new updated (decimal)value
change - absolute value of change (all negative numbers are being ignored)Update events value always by sending a difference (change to better. Example: when kills value goes from 11 to 12, you sends 1 (= the difference). When time of the best lap goes from 121.3 secs to 118.4 secs, send 2.9). Pay attention to correctness of these values.
-
EventAbs | GameArterSdk.I.EventAbs (eventNameId, absEventValue);
This function counts difference from previous value state itself and use recommended "Update" method on background. This function has same behaviour as recommended "Update" method, only is a bit slower.
Badges as a function of event updatesAn event can have associated badges (defined in Services configuration). By updating event value, there is automatical check for badges, and if certain event value is achieved, its badge (including asociated reward) is released automatically. Information about received badge is available also in the game, via "ReceivedBadges" function listener.
Leaderboard associated with an eventIf there is associated leaderboard with that event, SDK automatically updates also value of this event in the leaderboard. For quick manual push to leaderboard (e.g. at the end of brain game), there is recommended to use following helper function GameArterSdk.I.PostToLeaderboard (eventNameId, absEventValue);. By calling it, value of specified event is being updated and posted to leaderboard. This function is handy e.g. at the end of a brain game.
3.6. Accessing currency
There are more types of currencies in GameArter. The main currency is GameArter currency (GRT). This currency is available in all games running in FullSDK mode. Games running under LiteSDK mode have own local currency available in the game only. This local currency is convertible to GameArter currency (GRT) and vise versa under specific conversion ratios. This is mechanism protecting games in LiteSDK from affecting whole ecosystem by distributing and consuming too much of GRT currency. Exchange of the currencies is in a user-friendly form.
If you have own currency you want to use further in the game, you must make a mechanism which will guarantee parity of these two currencies. Only by this way, the currency can work properly. If you are not working on creating any mechanisms for currencies parity, you must use GameArter local currency as a default currency.
-
Get | GameArterSdk.I.LocalCurrency ();
returns amount of currency
-
Update | GameArterSdk.I.LocalCurrency (currencyChange);
var currencyChange = absGameCurrency - GameArterSdk.I.LocalCurrency(); GameArterSdk.I.LocalCurrency( currencyChange );
returns new amount of currency
-
(Currency) Exchange Module
Currency exchange is made via exchange module provided by SDK. The exchange can be opened by user's action from gameplayer, or by a call from the game. For opening exchange, call Garter.I.OpenSdkModule ("shop", (optional)callbackFunction);. Currency exchange is designed for currency exchange and increasing value of events with undefined trend.
Once a user leaves the exchange screen, SDK automatically returns information about closing the shop to callback function (for possibility to return game to state before opening it - e.g. unmute sounds) and current value of changed property (currency or event) to a function listening for "CurrencyUpdate" (in a case of currency) or "EventExternalUpdate" in a case of event. If the scene the exchange was opened from is displaying a value of changed property inside any UI, you need to update value displayed in such UI.
copy// Open exchange GameArterSdk.I.OpenSdkModule("shop", function(state){ switch(state){ case "opened": // mute music, do nothing ... break; case "closed": // unmute music, do nothing ... break; } }); function _CurrencyUpdate(value){ // Defined in point 3.2 console.log("_CurrencyUpdate: ", currencyValue); // update text of currency counter // If change of currencyValue will affect game story by any kind, // there is good idea to post data on server now } function _EventValueExtUpdate(eventName, eventVal){ // Defined in point 3.2 console.log("_EventValueExtUpdate: ", eventName, eventVal); // event "eventName" new value "eventVal" now. // Update UI, if necessary }
Behaviour during opened exchange can be set from optional callback function (states "opened" and "closed")
NOTE: If you see an empty space after calling GameArterSdk.I.LocalCurrency (currencyChange);, you did not add any packages for your project. Add some for your project id and version via Project panel.
-
Saving currency
Local currency is not synchronized during every change of its state. It is because of currency is connected with event values, progress and bought items. Therefore, local currency is synchronized together with all the other data. This prevents user from losing an individual unit from the mentioned units. If a user loses latest progress, he will lose currency, bought items, as well as progress made from the time of the last save. This means, there is no separated lose due to auto-saving system.
3.7 Game storage management
There is no need to care about whether an user is logged in or not. SDK solves data management for both. In general, to offer the best playing experience, all data should be saved in SDK (no directly in any browser based storage). If your game uses any services as currency, leaderboards or achievements, browser based storage is something you have to forget.
3.7.1 Getting data
GameArterSdk.I.GetData(key);SDK keeps all data synchronized in the game (saved is RAM memory) and on server. Thanks to this, there is no limit for getting data from the SDK. Data can be returned directly, or via a callback.
var myKeyData = GameArterSdk.I.GetData("my-key");
If no data for "key" found, "null" is returned.
3.7.2 Posting data
GameArterSdk.I.PostData(key,value,callback);Events, currency and progress cannot be part of the data structure (they are saved in the SDK data layer directly - see Events schema)
// post data without callback
GameArterSdk.I.PostData(key, value); // value may be of any datatype
// post data with callback
GameArterSdk.I.PostData(key, value, function(error, response){
if (error) {
console.error(error);
} else {
console.log (response);
}
});
SDK contains limit for post data requests. In general, there is enabled 8 post requests every 2 minutes. This frequency can be dynamically managed by SDK on basis of server load. If the limit is exceeded, SDK returns an error informing about that via callback (error attribute).
Requests aggregation: In a case you do more post requests with minimum delay, SDK automatically aggregates all the post requests and send them in one request. If the request is aggregated, you will get callback response => "aggregated"
On background, SDK automatically synchronizes data in game and server layer to keep active auto-managed services like leaderboard or achievements. If you wish to post your data via this auto-synchronisation (not recommended), you can post the data to SDK layer by following way:
// without callback
GameArterSdk.I.SetIndividualGameData< string >("key",dataToBeSaved);
// with callback
var postDataError = GameArterSdk.I.SetIndividualGameData("key",dataToBeSaved);
if (postDataError !== null) console.error(postDataError);
3.7.3 Deleting data
GameArterSdk.I.ClearDataKey(key, callback);-
1
Deleting data of a single key
copy// without callback GameArterSdk.I.ClearDataKey("key"); // with callback GameArterSdk.I.ClearDataKey("key", function(error,response){ });
-
2
Deleting all data (user account)
Clearing data might be used in one case only - restarting game. Game restart is possible in two cases:
-
User's request | GameArterSdk.I.ClearDataUserReq( function(err,res){} );
Send this request on basis of user's interaction. (A user requires to clear all his game data and start the game from beginning). After the call, there will be displayed a confirmation box by the SDK where the user will have to confirm his request.
If a user agrees, res === "ok" is returned. If a user rejects clearing, res === "rejected" is returned.
-
End of a game | Garter.I.ClearDataUserConfirm( function(err,res){} );
Send this request, when a game must be restarted because of a game design reason (=end of the game - negative money, death...)
Clearing data restarts game automatically. In editor, function Initialized() (point 3.2) is called and data are rewritten by default data. www.text == null). In a browser, whole page is reloaded.
NOTE: Currently, with clearing data, a user loses also all badges he got in the game. New SDK update will bring a possibility to select which badges should be removed and which not.
-
User's request | GameArterSdk.I.ClearDataUserReq( function(err,res){} );
3.8 Working with GameArter modules
Games are running in a GamePlayer containing various modules. These modules might be opened by user's direct action or by a call from a game. There is also possibility to customize game-behavior during the time modules are being opened. It is also possible to open all the modules from gameplayer. Inserting call to action buttons directly to a game increases opening these modules.
GameArter modules can be opened from a game by calling GameArterSdk.I.OpenSdkModule ("moduleName", function(state){} );
// With callback
GameArterSdk.I.OpenSdkModule("moduleName", function(state){
switch(state){
case "opened":
// ...
break;
case "closed":
// ...
break;
}
});
// Without callback
GameArterSdk.I.OpenSdkModule("moduleName");
List of GameArter modules
- Login GameArterSdk.I.OpenSdkModule("login", (optional)callback);
Opens login window. Login window is automatically called by the SDK at the beginning of a game (if a user is not logged in already). SDK opens login window also after pressing log in button in GameArter_Features prefab. Use this call only if a user is not logged in (check via .IsLoggedUser()) and by your own environment.
- Badges Garter.I.OpenSdkModule ("badge", (optional)callback);
Opens viewer of all badges available in the game. Received badges are visibly separated from other badges.
- Leaderboard Garter.I.OpenSdkModule ("leaderboard", (optional)callback);
Viewer of leaderboard state is being in progress, but you can add these calls already now. A user will be informed, that it will come soon. Once leaderboards viewer will be ready, it will begin work automatically.
- User's web profile Garter.I.OpenSdkModule ("profile", (optional)callback);
Opens user's web profile. This is the place a user can change his nick, image, or log out.
- Share Garter.I.OpenSdkModule ("share", (optional)callback);
Opens sharing window via which a user can share the game.
- Exchange Garter.I.OpenSdkModule ("exchange", (required)callback);
Opens an exchange. See more in point 3.7 - Currency exchange.
- Discussion Garter.I.OpenSdkModule ("discussion", (optional)callback);
Opens a discussion.
- Bug reporting Garter.I.OpenSdkModule ("report", (optional)callback);
Opens box for reporting bugs or any other feedback.
- Development Info Garter.I.OpenSdkModule ("development", (optional)callback);
Opens module with information about development. This is place with information about game updates. Such a small blog. There can be polls and so on.
- Video Garter.I.OpenSdkModule ("video", (optional)callback);
Opens box with a video. Videos to the module might be added via GameArter.com website.
- Game infobox Garter.I.OpenSdkModule ("gameinfo", (optional)callback);
Opens a window displaying game controls and Developer information.
- More games | Garter.I.OpenSdkModule ("moregames", (optional)callback);
Opens window with other recommended games to play.
3.9 Ads
Available ad types
- Fullscreen midroll ads (= Interstitial ads)
- Rewarded ads (= Fullscreen ads with a reward for watching)
Ad types can be combined in a game (One game can use both types of ads)
Fullscreen ad
Ad rules
- Call ads only in suitable places. Ads cannot affect best user experience (UX) from playing games
- Call ads in any suitable place for ad
- Game sounds MUST be muted during an ad
- Any exhortation or rewards for interacting with ads is prohibited
What is happening after an ad call:
- ad request is received in GameArter Ads manager.
- GameArter Ads manager checks availability of ads and processes ad-frequency-check.
- Ad state is returned by callback function.
Ad request
GameArterSdk.I.CallAd("midroll",function(adState){
switch(adState){
case "loaded":
// mute game
break;
case "completed":
// unmute game
break;
case "ignored":
// do nothing (it's beacuse the minimum time since the last advertisement has not been reached)
break;
default:
// all other ad statuses provided by ad provider (e.g. "started")
// these statuses have informatic character only.
}
});
Ads in loop
Best practices:
- First ad call from the start of the game. An ad at the beginning of the game does most of the income (the highest number of views). GameArter ad manager works with 2 types of preroll ads. In a case, that a website does not have own preroll, GameArter ad manager displayes an preroll ad on a load of gameplayer. In such case, first ad call from game is ignored. If no preroll on load of gameplayer is displayed (because it is not possible or is disabled), an ad on first ad call is displayed (= replacement of the preroll ad). From that reason, insert first ad call always at the beginning of the game.
Ad configuration for individual websites where the game is published is visible in Reports section after release of the game. There you can find out preroll ad type, frequency of ads, maximum length of ads, ad providers and so on. GameArter uses a mix of ad providers including own ads due to cover all websites restrictions (mainly policies for displaying Google Ads and ads.txt standard) and for achieving the best possible CPM. If you would like to make a change in default settings for a certain website or your game, contact GameArter support.
Rewarded ad
Availability of a rewarded ad is individual based on user's behaviour. Display button / option to launch rewarded ad only if the rewarded ad is available.
Callback states
- ignored = ad request has been ignored. Probably due to no ad (be sure you check ad availability before requests!)
- loaded = Rewarded ad has been loaded. Game sounts must be muted
- completed = An ad has been succesfully completed. Release reward + unmute sounds
- failed = something went wrong - user canceled the ad unmute sounds without reward
Implementation
if(GameArterSdk.I.RewardedAdAvailability()){
// display button / UI for requesting rewarded ad
}
// On rewarded ad request from user (e.g. pressing rewarded ad button)
GameArterSdk.I.CallAd("rewarded",function(adState){
switch(adState){
case "loaded":
// mute game
break;
case "completed":
// release reward
// unmute game
break;
case "failed":
// unmute game
break;
default:
// all other ad statuses provided by ad provider (e.g. "started")
// these statuses have informatic character only.
}
});
3.10 Analytics
You can use any tool for tracking you want. Do not you know any? Here's example of few of them.
By default, GameArter has implemented and supports Google Analytics for websites with a bridge of caching game-side data events. All you need to do to activate it, is to insert your own Google Analytics tracking id to "Basic settings" tab of your project. This analytics tracks all gameplayer-based data as players, source of visits, time of playing and so on. These data can be extended by data in a form of events sent from a game, see Google analytics guide page. Some basic events as scene change and so on can be send to analytics automatically on basis of analytics configuration.
Send any information you want to analytics by call:
GameArterSdk.I.AnalyticsEvent((string)action, (string)category, (string)label, (num)value);
/*
where:
category, label, value are optional parameters
See more at: https://developers.google.com/analytics/devguides/collection/analyticsjs/events
*/
3.11 Fullscreen
Because of other services around a game, fullscreen mode must be called over GameArterSDK. Attach following call to fullscreen button in your game (if your game has such button). If a game is already in fullscreen mode, fullscreen is cancelled by the call.
GameArterSdk.I.Fullscreen();
3.12 Redirects / Branding
-
In-game brand
SDK contains a multi-brand solution. In short, this feature allows to display all gameplayer logos on basis of a website the game is displayed on. Changes of outgoing links are also included. This feature is active for websites which promote games from GameArter system. With gameater promotion, there should be more gameplayes and higher incomes.
If there is any brand logo in the game, it should be connected to this system as well. Logo to display you can get on address GameArterSdk.I.BrandLogo(); and redirect can be processed by calling GameArterSdk.I.OpenWebPage();
-
Tracking redirects
With our solution you will know about every made redirect. - From what logo, on what website, and how oftenly is being used.
For tracking redirects, call GameArterSdk.I.OpenWebPage((string)targetUrl, (bool)sameWindow);
3.13 SDK cutscenes module
Every game contains many data-weight cutscenes, stories and animations which are displayed calmly only one time for the whole game. GSDK helps to reduce game filesizes by providing a story module — an option for downloading these stories from external storage only in a time when it’s required. Dynamic loading of game stories might be done via asset bundles or this SDK feature when the story is displayed in gameplayer above the game (like an ad).
-
1) Calling browser stories | GameArterSdk.I.RunCutScene ( animationNumber, function(state){} );
Be sure that animation of such number is available for your game.
-
2) Callback functions
There is a callback state - "not found","loaded", progress states and "completed".
- 3) Setting / switching of stories
Is being made in "More" section of configuration of the project
GameArterSdk.I.RunCutScene(0, function(progress){
switch(progress){
case "loaded":
// mute sounds, pause game
break;
case "completed":
// unmute sounds, unpause game
break;
case "not found":
// check that you have really devinned story with id 0
break;
default:
console.log("current story progress is:",progress);
}
});
3.14 Login during a game
If there is a log-in during a gameplay, SDK automatically re-initialize themselve by new data. Listen for .SdkInitialized event and set the game mechanism so to reinitialize whole the game by new data as well.
3.15 Game as App
GameArter SDK allows to installation of web games as Web Apps to user's device. Installation is possible to PC as well as mobile device.
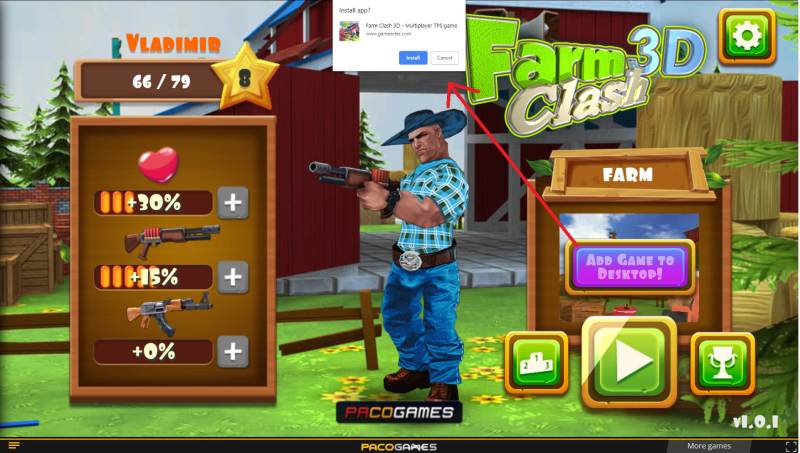
Implementation
- NOTE: this feature is functional after submiting requests for publishing in GameArter.
- Icon of web app (displayed at user's screen of desktop / mobile) must be in .png format and in 2 dimensions - 192x192 & 512x512px. Name them "favicon-192.png" and "favicon-512.png" and attach to folder "_pwa". Upload this folder with other project files in "Files upload" section at GameArter panel.
-
1) External callback listener | GameArterSdk.I.AddExternalCbListener("PWAState", targetFunction);
The listener is used for setting visibility of button "installing game as app". Design of the button is up to developer.
copyGameArterSdk.I.AddExternalCbListener("PWAState", InstallAsAppButton); function InstallAsAppButton(state){ switch(state){ case "enabled": // Display button break; case "disabled": // hide button break; } }
Button must be hidden in default.
- Next option, e.g. when a user comes to menu and there is not known state in the game →
Get current state value | GameArterSdk.I.GetStatePWA()
Returns "enabled" / "disabled". See point 1. -
2) Request for instalation
Request for adding game as app to user device is made by user's click at button "installing game as app". Returned state identifies about current state of installation. These states maz be sent to e.g. analytics for tracking click ratio.
copybuttonAddAsApp.onclick = InstallGameAsApp; function InstallGameAsApp(){ GameArterSdk.I.InstallAsPWA(function(state){ switch(state){ case "rejected": // request is rejected. Be sure the request is posted only if GameArterSdk.I.GetStatePWA() === "enabled". break; case "dismissed": // user cancelled the request break; case "accepted": // user accepted the request break; case "installed": // game was succesfully installed as app break; } }); }
Visibility change of button during these states is driven via listener for "PWAState", see point 1.
4. Ready to use UI
Ready to use UI elements saving development time. In progress.
5. Testing
Start your game. Most SDK services are also available in Unity editor. Before an upload, you must check:
- Functional communication with server.
The game has an access to server and can post and get data. If you need to clear all posted data, you can make it via button "Clear user's game data" in GameArter_Initialize object.
- Events, currency etc. are set and working properly
You do not have a mistake in working with events. Events contain correct values. Badges are returned in the right time.
All features are functional directly in editor as well as on web. In a case of external-opened window from editor, firstly make an action in the external web window, then press "close" button in the Unity UI informing you that "External window has been opened".
- When a module is opened, the game has corect behavior on background. Game must be muted with visible pointer during ads.
- All works as expected
6. Upload to GameArter
6.1. Space for uploading files
Game files must be uploated to GameArter via "files upload" section (button available in project panel). Every project version has own place for its game files.
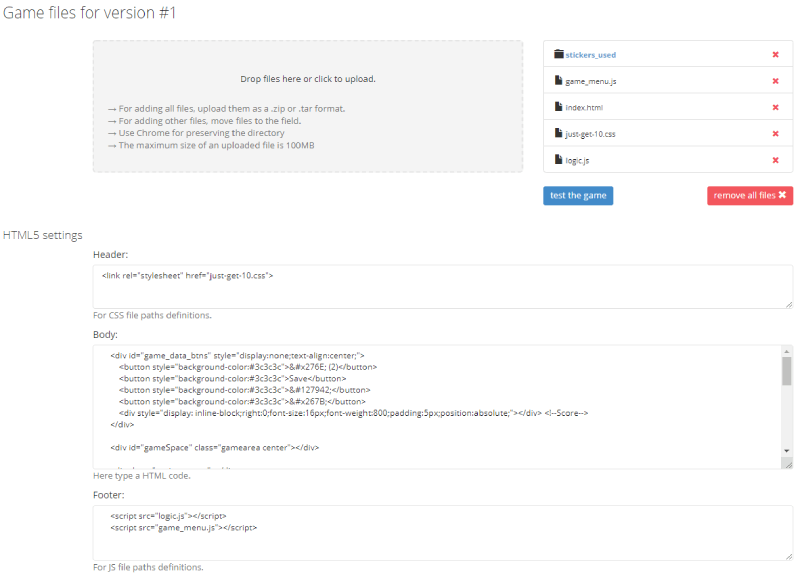
6.2. Working with files structure
Mechanism for uploading files is build above Dropzone Open Source library.
Uploading files may be done by dropping files in your PC and moving them to DropZone area in GameArter
If your browser does not allow simple move & drop files mechanism, eventually the upload does not work as expected (e.g. inner folders are ignored), there is still possible to upload complete files inserted in .zip file.
New uploaded files automatically replaces files of the same filename. Cache is being cleared automatically during every file load.
Test the game button is available only if you are in a folder containing file index.html file (must be uploaded)
There is no possible to insert external links to body section. Load 3rd party links and libraries dynamically, if needed.
6.3. Creating universal index.html file
GameArter uses own-created GamePlayers designed purely for targeted platforms (a game runs in different gameplayer on every platform). For simple multi-player management, "files upload" section contain three textarea elements. Top area is designed for placing styles, middle area for placing game body elements, and the bottom for script elements. Once these text areas are filled, and this change saved, GameArter's gameplayers are created automatically on basis of data filled in text areas for style, body and script elements.
GameArter styles and javascripts are placed into gameplayer automatically. Do not place them manually, manual insertion is only for testing on localhost. GameArter libraries are placed at the top of footer element, so be sure that all scripts comminicating with GameArter SDK are placed in the footer element.
6.4. Setting GamePlayer
In a tab GamePlayer you can customize gameplayer. You can fill there game description, controls, links to social sites and so on.
7. Game releases
Managed by "Publication" section of a project.
7.1 Piracy protection
Before uploading your game to GameArter platform and processing release, be sure your intelectual property in a form of written game is being protected.
Currently, GameArter works on automatical obfuscation of uploaded source codes, meanwhile till this will be processed automatically you can do it for free yourself online.
Source code Obfuscation is good protection from stealing game code. To protect stealing the game and uploading it at other platforms, there is recommended to extend obfuscated files about simple domain check, e.g:
try{
var allowedDomains = ["pacogames.com","gamearter.com"],
host = document.location.href.split("/")[2].split("."),
fullHost = host[host.length-2]+"."+host[host.length-1];
if(allowedDomains.indexOf(fullHost) > -1){
// run game
} else {
alert("Please, load the game from PacoGames.com");
document.getElementsByTagName("body")[0].innerHTML = "Unauthorized game start. Play the game on PacoGames";
}
} catch(e){
document.getElementsByTagName("body")[0].innerHTML = "Unauthorized game start. Play the game on PacoGames";
alert("Some kind of problem occured. Please, let us know about the problem at [email protected]");
}
7.2 First release
Once the game is ready to release, you can get a public link for free spreading in the "Publish" section of the project after pressing "Publish now button". In this case, game is automatically fully set and you can start share the game with players. In one working day since release, GameARter also will make additional check of the game and its functionality. Once the game is checked, you will be informed about result on Slack or email. If all is ok, the game will be sent to hundreds of webmasters in the system. Next day after release, the game will be displayed in reports sections of GameArter dashboard.
Important note: Once you press publish now button, the game passess from "testnet" to "release" state. In a case that your game is connected with GRT currency, any updates of events / items / packages will be visible in the game after confirmation from a side of administrators. This is a protection from manipulation with prices and affecting value of GRT token. Therefore be sure you are requesting release state when you are sure you will not update your released version of game. For testing next updates before release, you can use testnets where you can still edit all the items without any limitation.
7.3 Game updates
Game updates are fully managed by developers via Publication tab in project environment. (Project section at GameArter). After pressing "Publish now" button, previous game version is instantly replaced for a new game version automatically. By this action, you take full responsibility for the update so be sure the new version works well.
GameArter keeps 2 last game versions for option to return back in a case of need.
Game reviews (reqire up to 1 working day) and updates (processed immediatelly without any control by administrators) are made on basis of requests for updates / publishing. Do not send these request before you are sure that your game is fully functional.