Before you start
Every GameArter SDK mode is designed for specific user-cases. Basic SDK is the most simple SDK designed for very simple games or developers who are not interested in implementing advanced GameArter features to their games.
- Continue only if you meet following points:
- The game does not contain user profiles.
- The game does not save any user data on server.
Estimated time of SDK implementation: 30 minutes. Please, follow the guide carefully, it will save you from many future problems.
1. Getting started
At the very beginning, there is need to set basic project configuration.
1.1. Download SDK package
Download latest version of GameArter SDK Unity package from GameArter SDK for HTML5 Package section.
1.2. Import GameArter SDK package to the game project
Importing GameArter SDK allows full work with SDK in own index.html file (editor mode) running on address file:// directly in the pc.
Importing GameArter's SDK consist of placing .css and .js file to index.html file.
After uploading final version to server, the index.html file will be automatically replaced for full GameArter's GamePlayer.
Be sure, GameArter's .css and .js file do not affect any game javascript or css (Only reserved var is "Garter"). Right import of GameArter's SDK require to keep following structure of index.html file:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<!-- Editor GamePlayer for testing (it will be automatically replaced after upload to gamearter) -->
<link rel="stylesheet" media="(min-width:480px)" href="https://www.gamearter.com/css/style-gp.css">
<link rel="stylesheet" media="(max-width:480px)" href="https://www.gamearter.com/css/mobile-wrapper.css">
<!-- GAME STYLES SPACE START-->
// Space for game styles
<!-- GAME STYLES SPACE END-->
</head>
<body>
<main role="main" id="main" class="main" style="height:100%;padding-left:0">
<div id="ga_game" style="height:100%;;position:relative">
<!------- GAME BODY SPACE START --------->
// Space for game body
<!--------- GAME BODY SPACE END ---------->
</div>
</main>
<footer role="contentinfo" class="footer-gp no-select">
<img src="https://data.pacogames.com/gplayer/branding/gamearter-uni.png" alt="Pacogames" width="184" height="18">
<a href="https://developers.gamearter.com/projects">Project Panel</a> |
<a href="https://developers.gamearter.com/docs/html5/sdk-overview.php">SDK Documentation</a>
</footer>
</body>
<script src="https://www.gamearter.com/gameplayer/html5/gamearter-gameplayer.js"></script>
<script src="https://www.gamearter.com/gameplayer/html5/gamearter-instance.js"></script>
<!-- GAME SCRIPTS SPACE START -->
// Space for game scripts
<!-- GAME SCRIPTS SPACE END -->
</html>
You can modify your own index.html file or extend following player prefabs - desktop-gameplayer.html, mobile-gameplayer.html - about your game lines.
In production, GameArter automatically run desktop or mobile gameplayer on basis of targeted device. This allow to deliver the best possible experience for these devices.
1.3. Fixing possible errors
- Game framework does not allow implementation of GameArter SDK by this way | Let us know your game framework / engine and we will make an extension of GameArter SDk for this framework / engine, if possible.
- Broken styling | This maight be affected either by a clash in GameArter's style with game style. There may be also different default style settings for running from localhost and server.
- No game displayed | Be sure that canvas (or game div) is displayed in visible area of screen. Game div must be inside GameArter's div element with id "ga_game".
- Need a help?GameArter Slack: @adm_vladimir / email - [email protected] /
2. SDK configuration
Before a possibility to connect to the SDK, there is need to make a project configuration.
2.1. Create a project
Create new html5 game project in projects section of GameArter system.
This is way you will get your project Id for communication with GameArter SDK.
2.2. Project configuration
Create a configuration for selected project version. You can have up to 5 versions of every project. (Only 1 version can be live, the other 4 serves for development purposes or AB testing).
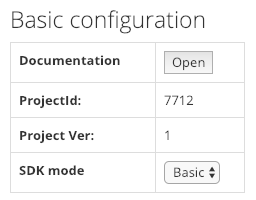
-
1
Documentation
A button opening knowledge base you are currently on. -
2
Project ID
Every game must have its unique project ID. Project ID is a signature of the game with which it accesses, the game has access to GameaArter features. -
3
Project Ver
Selected project version you are configurating -
4
SDK mode
The mode, under which the SDK will run. Select Basic.
Remember you projectId and Project Ver for next steps. Once done, save the basic configuration by pressing "Save configuration"
2.3. Services configuration
Services configuration is for files management, project versions management and adjusting data filled in the project configuration and for adding additinal SDK features in higher modes of GameArter SDK.
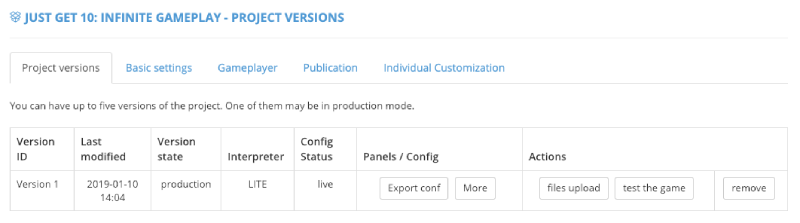
- Rows / Buttons explanation:
- Version ID | project version
- Last modified | Last modification in the version configuration
- Version State | project mode - testnet / production
- Interpreter | interpreter under which the project version runs
- Config status | Status of data state - draft / pending (= waiting for authorization by admins) / live (= approved,in production)
- Export conf buttonn | Opens project configuration (panel 2.2)
- More button | Opens configuraion of additional features
- Files upload button | Opens environment for uploading game files
- Test the game button | Opens game in a new tab
- Remove button | Removes project version
3. Working with SDK
3.1. SDK initialization
For possibility to connect and use GameArter SDK, there is need to create a new GameArter SDK instance.
var GameArterSdk = new GamearterInstance({
projectId:0, // Insert your project id here
projectVersion:1, // Insert your project version here
sdkMode:"B", // Use SDK in Basic mode
sdkVersion:"2.0" // use SDK ver 2.0
}, function(err){ if(err) console.error(err); } );
Callback function (attribute 2) is optional parameter via which you can be informed about errors occured during SDK initialization (e.g. SDK is already initialized, different projectId than expected projectId ... ).
During initialization, by adding other parametres there is possible to adjust SDK behavior. Following table display other options:
System options
key | value | default value | description |
---|---|---|---|
notifications | boolean | true | Displayes notification related to SDK ("Loading data", "saving data", "Badge XY received" ...) in top left corner. |
editor options
key | value | default value | description |
---|---|---|---|
debug | boolean | true | Prints additional informations to console |
prerollAd | boolean | false | Activates preroll ad |
minAdTime | num [miliseconds] | 1000 | Sets minimum time between ads to GameArter's ad manager. (1000 = 1s) |
Example of creating SDK instance with additional options:
var GameArterSdk = new GamearterInstance({
projectId:0, // Insert your project id here
projectVersion:1, // Insert your project version here
sdkMode:"B", // Use SDK in Basic mode
sdkVersion:"2.0", // use SDK ver 2.0
notifications: true, // enable SDK notification
developmentMode: {
debug: false, // do not print to console
prerollAd: true, // display preroll ad
minAdTime: 60000 // set minimum time between ads at 1 minute
}
}, function(err){ if(err) console.error(err); } );
At the example above, SDK functions are available on path GameArterSdk.I. ... . Rest of the documentation will work with GameArterSdk as the var of GameArter instance.
3.2. SDK Listeners installation
In general, listeners are processed by callback function of calls made to the SDK. This sections is related to listeners for external events like SDK initialization or rewards to player (defined on server, not in the game).
Installation of listeners
GameArterSdk.I.AddExternalCbListener(A, B, C );
where:
- A: Event the listener is set for
- B: Function into which is sent information once the event occur
- C: Callback function informing about success / fail (optional)
Available external listeners
eventKey | attribute value | description |
---|---|---|
SdkInitialized | - | It is called once GameArter SDK is fully initialized (all calls and services are available) |
PossibleGameExit | - | It's called when SDK recognizes risk of escaping the game (e.g. closing page tab). This may be last opportunity to save game porgress. |
PWAState | - | Progressive Web App state. It's called when progressive web app state is being changed. -> enabled = display install game as app button | -> disabled = hide install game as app button. Button install game as app must be hidden in default. See more in section 3.15 - Game as App. |
Example of listeners installation for external SDK events
// Listeners
GameArterSdk.I.AddExternalCbListener("SdkInitialized", _GameArterSdkInitialized, function(err,res){ console.log(err,res); });
GameArterSdk.I.AddExternalCbListener("PossibleGameExit", _PossibleGameExit, function(err,res){ console.log(err,res); });
GameArterSdk.I.AddExternalCbListener("PWAState", _PwaState, function(err,res){ console.log(err,res); });
// Listeners functions
function _PossibleGameExit(){ console.log("_PossibleGameExit: ", state); }
function _GameArterSdkInitialized(){ console.log("--- SDK INITIALIZED !!! -→ load the game ---"); }
function _PwaState(state){ console.log("PWA:",state); }
NOTE: From security reasons, GameArterSdk must be used within a scope (e.g. in function, class, or prototype) to prevent its global accessability.
Possible start implementation:
- Screen / box "Loading"
- Wait for "SdkInitialized" event.
- Set game - data, UI...
- Remove "Loading screen (point 1) and let a user play"
3.3. Accessing SDK data
-
1
Individual Game Mode
There's a possibility you require to run the game in individual game mode on certain website or e.g. for testing. By using individual game mode features, you can set individual mode the game will run in for every website the game is published on. SDK will return you after its initialization number of mode which you will assign to a certain website. Then, you can simply set data with which the game should run according the mode number.
Get mode number under which the game should run:
var individualGameModeNumber = GameArterSdk.I.IndividualGameMode(); -
2
Browser name
WebGL games have often different performance in various browsers. With knowledge of a browser a game is running in, there is a possibility for optimization of disabling features for certain browsers. E.g. car destructions for Chrome.
var browserName = GameArterSdk.I.GetBrowserName();
3.4. Getting user info
On SDK initialized state, you can start any interaction with SDK.
GameArter has implemented auto-login and auto-authentication system. There is always returned a user identity even when a game does not use login system.
var userNick = GameArterSdk.I.UserNick(); // user's nickname or Guest#randomNumber
var userLang = GameArterSdk.I.UserLang(); // default language a user has set on pacogames // ar, cs, de, en, es, fr, pl, pt, ru, tr
var userCountry = GameArterSdk.I.UserCountry(); // country shortcut the user is connected from
var loggedUser = GameArterSdk.I.IsLoggedUser(); // returns true, if user is logged in
var userImageUrl = GameArterSdk.I.UserImage (); // returns user's profile img as a texture () [1]);
var userRankName = GameArterSdk.I.UserRankName(); // returns profile rank of a user
3.5. Ads
Available ad types
- Fullscreen midroll ads (= Interstitial ads)
- Rewarded ads (= Fullscreen ads with a reward for watching)
Ad types can be combined in a game (One game can use both types of ads)
Fullscreen ad
Ad rules
- Call ads only in suitable places. Ads cannot affect best user experience (UX) from playing games
- Call ads in any suitable place for ad
- Game sounds MUST be muted during an ad
- Any exhortation or rewards for interacting with ads is prohibited
What is happening after an ad call:
- ad request is received in GameArter Ads manager.
- GameArter Ads manager checks availability of ads and processes ad-frequency-check.
- Ad state is returned by callback function.
Ad request
GameArterSdk.I.CallAd("midroll",function(adState){
switch(adState){
case "loaded":
// mute game
break;
case "completed":
// unmute game
break;
case "ignored":
// do nothing (it's beacuse the minimum time since the last advertisement has not been reached)
break;
default:
// all other ad statuses provided by ad provider (e.g. "started")
// these statuses have informatic character only.
}
});
Ads in loop
Best practices:
- First ad call from the start of the game. An ad at the beginning of the game does most of the income (the highest number of views). GameArter ad manager works with 2 types of preroll ads. In a case, that a website does not have own preroll, GameArter ad manager displayes an preroll ad on a load of gameplayer. In such case, first ad call from game is ignored. If no preroll on load of gameplayer is displayed (because it is not possible or is disabled), an ad on first ad call is displayed (= replacement of the preroll ad). From that reason, insert first ad call always at the beginning of the game.
Ad configuration for individual websites where the game is published is visible in Reports section after release of the game. There you can find out preroll ad type, frequency of ads, maximum length of ads, ad providers and so on. GameArter uses a mix of ad providers including own ads due to cover all websites restrictions (mainly policies for displaying Google Ads and ads.txt standard) and for achieving the best possible CPM. If you would like to make a change in default settings for a certain website or your game, contact GameArter support.
Rewarded ad
Availability of a rewarded ad is individual based on user's behaviour. Display button / option to launch rewarded ad only if the rewarded ad is available.
Callback states
- ignored = ad request has been ignored. Probably due to no ad (be sure you check ad availability before requests!)
- loaded = Rewarded ad has been loaded. Game sounts must be muted
- completed = An ad has been succesfully completed. Release reward + unmute sounds
- failed = something went wrong - user canceled the ad unmute sounds without reward
Implementation
if(GameArterSdk.I.RewardedAdAvailability()){
// display button / UI for requesting rewarded ad
}
// On rewarded ad request from user (e.g. pressing rewarded ad button)
GameArterSdk.I.CallAd("rewarded",function(adState){
switch(adState){
case "loaded":
// mute game
break;
case "completed":
// release reward
// unmute game
break;
case "failed":
// unmute game
break;
default:
// all other ad statuses provided by ad provider (e.g. "started")
// these statuses have informatic character only.
}
});
3.6. Analytics
You can use any tool for tracking you want. Do not you know any? Here's example of few of them.
By default, GameArter has implemented and supports Google Analytics for websites with a bridge of caching game-side data events. All you need to do to activate it, is to insert your own Google Analytics tracking id to "Basic settings" tab of your project. This analytics tracks all gameplayer-based data as players, source of visits, time of playing and so on. These data can be extended by data in a form of events sent from a game, see Google analytics guide page. Some basic events as scene change and so on can be send to analytics automatically on basis of analytics configuration.
Send any information you want to analytics by call:
GameArterSdk.I.AnalyticsEvent((string)action, (string)category, (string)label, (num)value);
/*
where:
category, label, value are optional parameters
See more at: https://developers.google.com/analytics/devguides/collection/analyticsjs/events
*/
3.7. Fullscreen
Because of other services around a game, fullscreen mode must be called over GameArterSDK. Attach following call to fullscreen button in your game (if your game has such button). If a game is already in fullscreen mode, fullscreen is cancelled by the call.
GameArterSdk.I.Fullscreen();
3.8. Redirects / Branding
-
In-game brand
SDK contains a multi-brand solution. In short, this feature allows to display all gameplayer logos on basis of a website the game is displayed on. Changes of outgoing links are also included. This feature is active for websites which promote games from GameArter system. With gameater promotion, there should be more gameplayes and higher incomes.
If there is any brand logo in the game, it should be connected to this system as well. Logo to display you can get on address GameArterSdk.I.BrandLogo(); and redirect can be processed by calling GameArterSdk.I.OpenWebPage();
-
Tracking redirects
With our solution you will know about every made redirect. - From what logo, on what website, and how oftenly is being used.
For tracking redirects, call GameArterSdk.I.OpenWebPage((string)targetUrl, (bool)sameWindow);
3.9. Cutscenes module
Every game contains many data-weight cutscenes, stories and animations which are displayed calmly only one time for the whole game. GSDK helps to reduce game filesizes by providing a story module — an option for downloading these stories from external storage only in a time when it’s required. Dynamic loading of game stories might be done via asset bundles or this SDK feature when the story is displayed in gameplayer above the game (like an ad).
-
1) Calling browser stories | GameArterSdk.I.RunCutScene ( animationNumber, function(state){} );
Be sure that animation of such number is available for your game.
-
2) Callback functions
There is a callback state - "not found","loaded", progress states and "completed".
- 3) Setting / switching of stories
Is being made in "More" section of configuration of the project
GameArterSdk.I.RunCutScene(0, function(progress){
switch(progress){
case "loaded":
// mute sounds, pause game
break;
case "completed":
// unmute sounds, unpause game
break;
case "not found":
// check that you have really devinned story with id 0
break;
default:
console.log("current story progress is:",progress);
}
});
3.10 Game as App
GameArter SDK allows to installation of web games as Web Apps to user's device. Installation is possible to PC as well as mobile device.
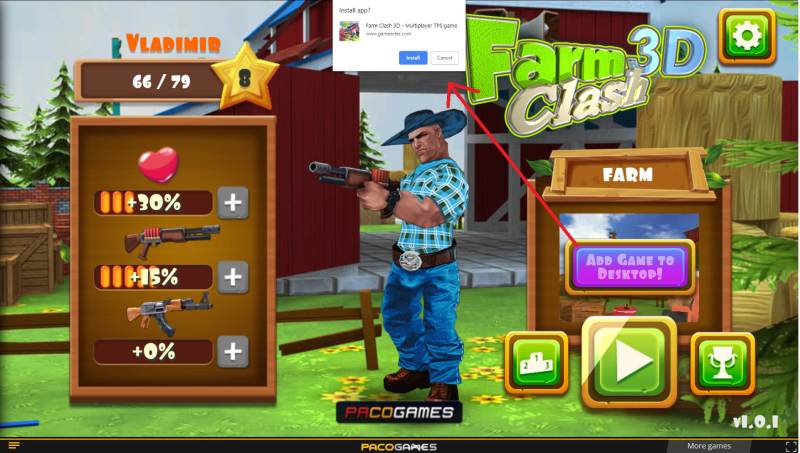
Implementation
- NOTE: this feature is functional after submiting requests for publishing in GameArter.
- Icon of web app (displayed at user's screen of desktop / mobile) must be in .png format and in 2 dimensions - 192x192 & 512x512px. Name them "favicon-192.png" and "favicon-512.png" and attach to folder "_pwa". Upload this folder with other project files in "Files upload" section at GameArter panel.
-
1) External callback listener | GameArterSdk.I.AddExternalCbListener("PWAState", targetFunction);
The listener is used for setting visibility of button "installing game as app". Design of the button is up to developer.
copyGameArterSdk.I.AddExternalCbListener("PWAState", InstallAsAppButton); function InstallAsAppButton(state){ switch(state){ case "enabled": // Display button break; case "disabled": // hide button break; } }
Button must be hidden in default.
- Next option, e.g. when a user comes to menu and there is not known state in the game →
Get current state value | GameArterSdk.I.GetStatePWA()
Returns "enabled" / "disabled". See point 1. -
2) Request for instalation
Request for adding game as app to user device is made by user's click at button "installing game as app". Returned state identifies about current state of installation. These states maz be sent to e.g. analytics for tracking click ratio.
copybuttonAddAsApp.onclick = InstallGameAsApp; function InstallGameAsApp(){ GameArterSdk.I.InstallAsPWA(function(state){ switch(state){ case "rejected": // request is rejected. Be sure the request is posted only if GameArterSdk.I.GetStatePWA() === "enabled". break; case "dismissed": // user cancelled the request break; case "accepted": // user accepted the request break; case "installed": // game was succesfully installed as app break; } }); }
Visibility change of button during these states is driven via listener for "PWAState", see point 1.
4. Ready to use UI
Ready to use UI elements saving development time. In progress.
5. Testing
Start your game. During ad calls, your game should be paused, without mouse. During opened modules, the game should be in the settings you set. (Paused and muted by default). Calls to analytics are visible in the console. If everything is right, you can build the project and go to the next section.
6. Upload to GameArter
6.1. Space for uploading files
Game files must be uploated to GameArter via "files upload" section (button available in project panel). Every project version has own place for its game files.
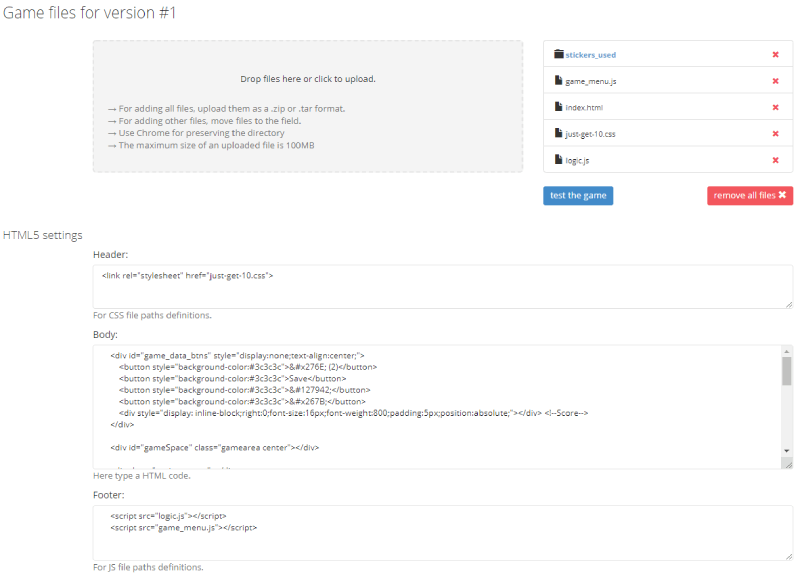
6.2. Working with files structure
Mechanism for uploading files is build above Dropzone Open Source library.
Uploading files may be done by dropping files in your PC and moving them to DropZone area in GameArter
If your browser does not allow simple move & drop files mechanism, eventually the upload does not work as expected (e.g. inner folders are ignored), there is still possible to upload complete files inserted in .zip file.
New uploaded files automatically replaces files of the same filename. Cache is being cleared automatically during every file load.
Test the game button is available only if you are in a folder containing file index.html file (must be uploaded)
There is no possible to insert external links to body section. Load 3rd party links and libraries dynamically, if needed.
6.3. Creating universal index.html file
GameArter uses own-created GamePlayers designed purely for targeted platforms (a game runs in different gameplayer on every platform). For simple multi-player management, "files upload" section contain three textarea elements. Top area is designed for placing styles, middle area for placing game body elements, and the bottom for script elements. Once these text areas are filled, and this change saved, GameArter's gameplayers are created automatically on basis of data filled in text areas for style, body and script elements.
GameArter styles and javascripts are placed into gameplayer automatically. Do not place them manually, manual insertion is only for testing on localhost. GameArter libraries are placed at the top of footer element, so be sure that all scripts comminicating with GameArter SDK are placed in the footer element.
6.4. Setting GamePlayer
In a tab GamePlayer you can customize gameplayer. You can fill there game description, controls, links to social sites and so on.
7. Game releases
Managed by "Publication" section of a project.
7.1 Piracy protection
Before uploading your game to GameArter platform and processing release, be sure your intelectual property in a form of written game is being protected.
Currently, GameArter works on automatical obfuscation of uploaded source codes, meanwhile till this will be processed automatically you can do it for free yourself online.
Source code Obfuscation is good protection from stealing game code. To protect stealing the game and uploading it at other platforms, there is recommended to extend obfuscated files about simple domain check, e.g:
try{
var allowedDomains = ["pacogames.com","gamearter.com"],
host = document.location.href.split("/")[2].split("."),
fullHost = host[host.length-2]+"."+host[host.length-1];
if(allowedDomains.indexOf(fullHost) > -1){
// run game
} else {
alert("Please, load the game from PacoGames.com");
document.getElementsByTagName("body")[0].innerHTML = "Unauthorized game start. Play the game on PacoGames";
}
} catch(e){
document.getElementsByTagName("body")[0].innerHTML = "Unauthorized game start. Play the game on PacoGames";
alert("Some kind of problem occured. Please, let us know about the problem at [email protected]");
}
7.2 First release
Once the game is ready to release, you can get a public link for free spreading in the "Publish" section of the project after pressing "Publish now button". In this case, game is automatically fully set and you can start share the game with players. In one working day since release, GameARter also will make additional check of the game and its functionality. Once the game is checked, you will be informed about result on Slack or email. If all is ok, the game will be sent to hundreds of webmasters in the system. Next day after release, the game will be displayed in reports sections of GameArter dashboard.
Important note: Once you press publish now button, the game passess from "testnet" to "release" state. In a case that your game is connected with GRT currency, any updates of events / items / packages will be visible in the game after confirmation from a side of administrators. This is a protection from manipulation with prices and affecting value of GRT token. Therefore be sure you are requesting release state when you are sure you will not update your released version of game. For testing next updates before release, you can use testnets where you can still edit all the items without any limitation.
7.3 Game updates
Game updates are fully managed by developers via Publication tab in project environment. (Project section at GameArter). After pressing "Publish now" button, previous game version is instantly replaced for a new game version automatically. By this action, you take full responsibility for the update so be sure the new version works well.
GameArter keeps 2 last game versions for option to return back in a case of need.
Game reviews (reqire up to 1 working day) and updates (processed immediatelly without any control by administrators) are made on basis of requests for updates / publishing. Do not send these request before you are sure that your game is fully functional.